Play Audio Array
This write up is mostly so I don't forget about this later on, but maybe fun for anyone using unreal audio.
I made a whole lot of narrator audio files for my project and batch converted them from mp3 to wav, then noticed that the converter (Reaper) by default adds a Tail (1000 MS silence at the end of each). This is fine; I just didn't notice, so when the sounds are concatenated I was getting gaps ... so i got chatgpt to write a pythonscript to remove the trailing silence at the end of the WAVs in the folder, all at once. Boom, done.
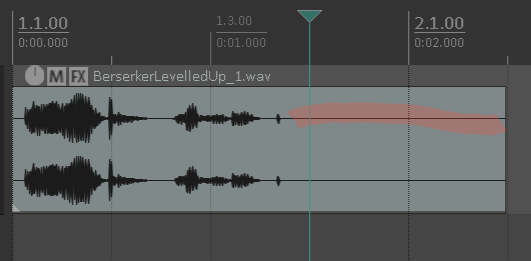
Well, in the first case I tried removing the 1000 ms Tail from the end, but it didn't work great, so instead I went for removing trailing silence, which worked better. However, in this case, I was annoyed because the Tail in ms is more accurate (for the problem) than detecting silence; however, besides the added Tail, my edits may have had even more silence on the end due to editing lots of files, so it's okay.

To use the script below, find the line folder_path = r"D:\Dropbox\UnrealBackups\Audio\Narrator" and replace it with the path to your folder of WAVs (that you need the silence at the end removed from). Note: this uses Silence Theshold and Min Silence Length values that are suggested ; seems to work fine though. Make a backup of your folder first though, just in case. Before running this, make sure your python install includes -pip install pydub
Python:RemoveWavTail
import os
from pydub import AudioSegment
from pydub.silence import detect_nonsilent
def main():
# Set your folder path here
folder_path = r"D:\Dropbox\UnrealBackups\Audio\Narrator"
# Adjust these values as needed:
# - silence_thresh (in dB)
# - min_silence_len (in ms) - the minimum length to be considered silence
silence_thresh = -50
min_silence_len = 200
# Create "Processed" subfolder if it doesn't exist
processed_folder = os.path.join(folder_path, "Processed")
os.makedirs(processed_folder, exist_ok=True)
for file_name in os.listdir(folder_path):
if file_name.lower().endswith(".wav"):
file_path = os.path.join(folder_path, file_name)
audio = AudioSegment.from_wav(file_path)
# Use pydub's silence detection to find all non-silent ranges
non_silent_ranges = detect_nonsilent(
audio,
min_silence_len=min_silence_len,
silence_thresh=silence_thresh
)
if not non_silent_ranges:
# If the file is entirely silent, optionally skip or create a zero-length file
print(f"Skipping (entirely silent): {file_name}")
continue
# Each element in non_silent_ranges is [start_ms, end_ms]
# We'll trim at the end of the last non-silent segment
end_of_last_segment = non_silent_ranges[-1][1]
# Keep from the beginning to the end of the last non-silent segment
trimmed_audio = audio[:end_of_last_segment]
# Export trimmed file to the "Processed" folder
processed_path = os.path.join(processed_folder, file_name)
trimmed_audio.export(processed_path, format="wav")
print(f"Processed (removed trailing silence): {file_name}")
if __name__ == "__main__":
main()
Then I bungled together a ForLoopWithDelay macro using the audio Duration of the next cue to play.

Then I made a SoundCue concatenator that plays an array of clips with the proper duration of each. The use case here is for if you want to do something like "{PlayerName} sold {#} rings for {#} gold!", but using a set of soundcues that join together.
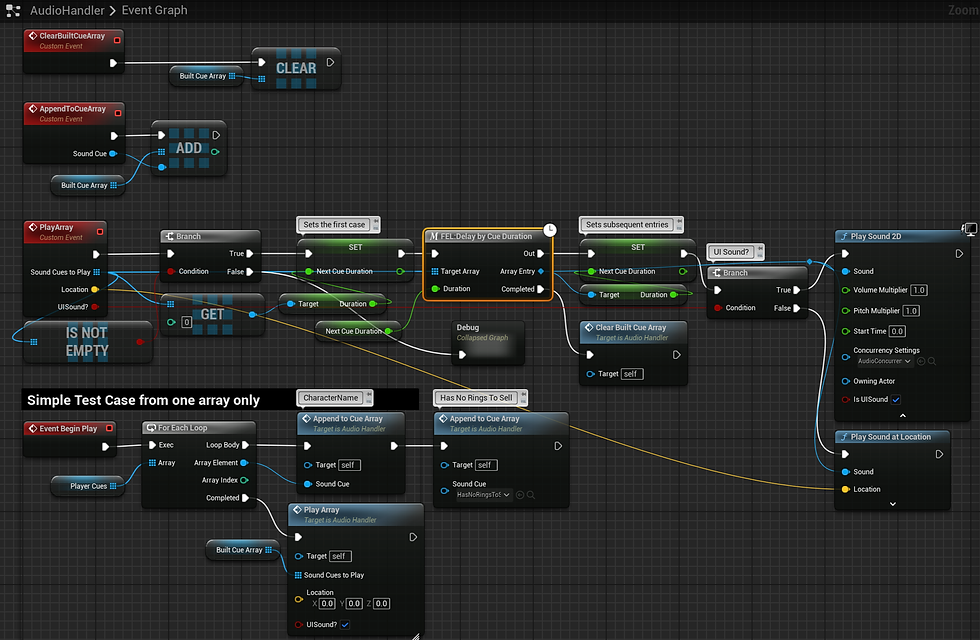
Note that for each SoundCue Output, unreal does a shifty thing where Volume Multiplier defaults to 0.75 Somewhere I wrote a batch to set all selected Cues to use 1.0 for volume so as to be true to the input WAV: https://tomofnz.wixsite.com/tomofnz/single-post/set-soundcue-volume-to-1-0-on-selected-assets Also, it's good to make sure the Sound Class of the cue is 'Voice' so that all voice cues have the same treatment when, for example, the player sets preferences for how loud Voice plays relative to Music or whatnot.
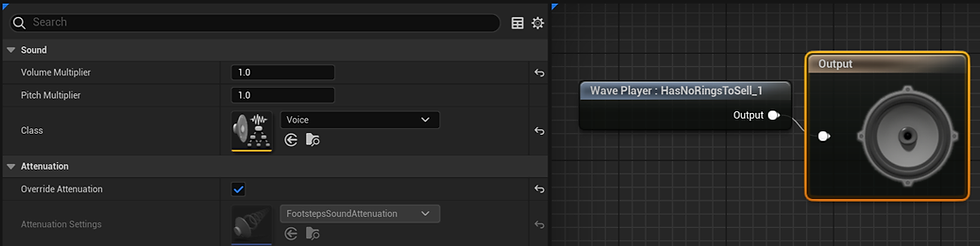
Comments